Free ways to view, create, and edit PDFs in PHP
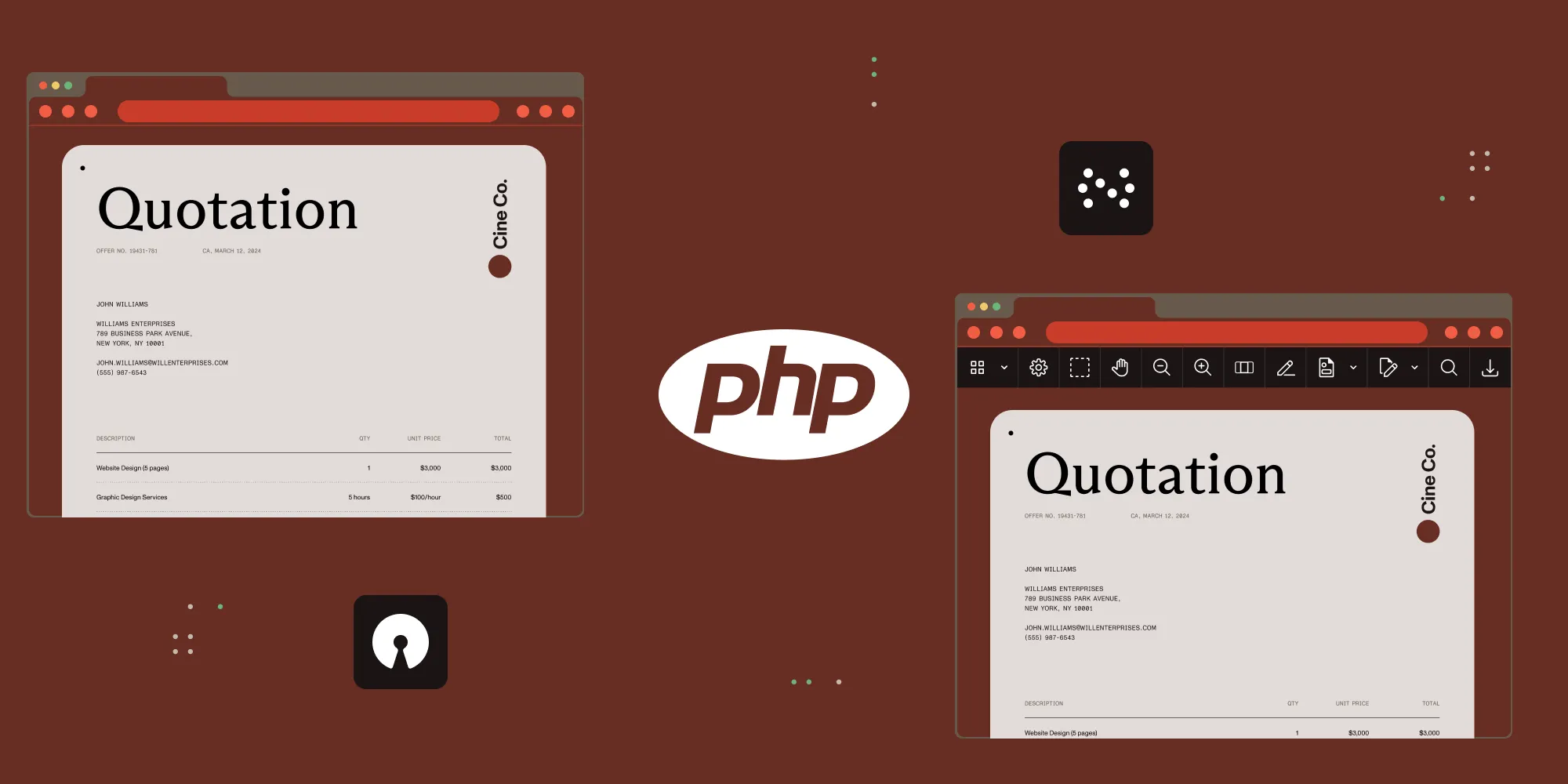
As developers, we often encounter the need to work with PDF documents within our PHP applications. Whether it’s displaying reports, generating invoices, or manipulating existing files, handling PDFs is crucial. The good news is that the PHP ecosystem offers a wealth of free and open source libraries to tackle these tasks.
This blog post will explore practical ways to integrate PDF functionality into your PHP projects. It’ll cover everything from simple PDF viewing in the browser, to generating and making structural edits using powerful libraries.
Leveraging the browser’s built-in PDF viewer
The simplest way to display a PDF in a PHP web application is to rely on the browser’s native PDF viewer. Modern browsers such as Chrome, Firefox, Edge, and Safari come equipped with this functionality out of the box.
How it works with PHP
PHP serves the PDF file with the correct headers. You can create direct links to your PDF files, and the browser will handle the rendering.
Requirements
- A web server running PHP. You can also run PHP’s built-in server using -
php -S localhost:8000
. - PDF files you want to display, accessible by your PHP script.
Step-by-step instructions
- Store your PDF files — Place your PDF files in a directory accessible by your web server (for example, a folder named
pdfs
in your web root). - Create a PHP page to list the PDFs (optional but useful) — You can create a PHP page that dynamically lists the available PDF files:
<!DOCTYPE html><html><head> <title>PDF viewer</title></head><body> <h1>Available PDF documents</h1>
- <a href="' . $filePath . '" target="_blank">' . htmlspecialchars($fileName) . '</a>
</body></html>
- Link directly to the PDF file — In your HTML, you can create a link to the PDF file. The
target="_blank"
attribute will open the PDF in a new tab, utilizing the browser’s built-in viewer:
[View my document](pdfs/my_document.pdf)
Embedding a JavaScript PDF viewer
For more control over the viewing experience — for example, a custom user interface (UI), or embedded viewing within your page — you can use a free JavaScript PDF viewer library.
Requirements
- A web server running PHP. You can also run PHP’s built-in server using -
php -S localhost:8000
. - PDF files that you want to display.
- A free JavaScript PDF viewer library (for example, PDF.js or other open source libraries).
Step-by-step instructions (using PDF.js as an example)
- Download PDF.js — Download PDF.js from its official repository and include it in your project. However, to integrate, you can often use a publicly hosted content delivery network (CDN) link. A reliable option is often provided by jsDelivr(opens in a new tab):
<script src="https://cdn.jsdelivr.net/npm/pdfjs-dist@latest/build/pdf.min.js"></script><script src="https://cdn.jsdelivr.net/npm/pdfjs-dist@latest/build/pdf.worker.min.js"></script>
Make sure to include both pdf.min.js
and pdf.worker.min.js
. You might need to adjust the @latest
tag to a specific version for more stability in production.
- Create an HTML page with a container for the viewer, as demonstrated in the code snippet below:
<!DOCTYPE html><html> <head> <title>PDF viewer</title> <style> #pdf-container { width: 80%; height: 600px; /* Adjust as needed */ margin: 0 auto; border: 1px solid #ccc; } </style> </head> <body> <h1>Embedded PDF viewer</h1> <div id="pdf-container"></div>
<script src="https://mozilla.github.io/pdf.js/build/pdf.js"></script> <script src="https://mozilla.github.io/pdf.js/build/pdf.worker.js"></script> <script> // The URL of the PDF file (can be dynamically generated by PHP). const pdfUrl = 'pdfs/another_document.pdf';
// Configure the worker path. pdfjsLib.GlobalWorkerOptions.workerSrc = `https://mozilla.github.io/pdf.js/build/pdf.worker.js`;
// Load the PDF. pdfjsLib.getDocument(pdfUrl).promise.then((pdf) => { // Fetch the first page. pdf.getPage(1).then((page) => { const canvas = document.createElement('canvas'); const context = canvas.getContext('2d'); const viewport = page.getViewport({ scale: 1.5 }); // Adjust scale as needed
canvas.height = viewport.height; canvas.width = viewport.width;
// Append the canvas to the container. document .getElementById('pdf-container') .appendChild(canvas);
// Render the page. page.render({ canvasContext: context, viewport: viewport, }); }); }); </script> </body></html>
- Serve the PDF file — Ensure the PDF file specified in the
pdfUrl
variable is accessible by the web server. You can use PHP to dynamically set this URL if needed:
<?php$pdfFile = 'pdfs/another_document.pdf';?><!DOCTYPE html><html><head> <title>PDF viewer</title> <style> /* ... CSS ... */ </style></head><body> <h1>Embedded PDF viewer</h1> <div id="pdf-container"></div>
<script src="https://mozilla.github.io/pdf.js/build/pdf.js"></script> <script src="https://mozilla.github.io/pdf.js/build/pdf.worker.js"></script> <script> const pdfUrl = '<?php echo htmlspecialchars($pdfFile); ?>'; // Configure the worker path (ensure this matches where `pdf.worker.js` is). pdfjsLib.GlobalWorkerOptions.workerSrc = `https://mozilla.github.io/pdf.js/build/pdf.worker.js`; // ... (the rest of the JavaScript code from step 2) ... </script></body></html>
Generating and manipulating PDFs with open source PHP libraries
There are open source PHP libraries that enable you to generate, create, and — to some extent — edit PDF files. However, the editing capabilities of most free PHP libraries are usually focused on manipulating existing PDF structure — such as merging, splitting, adding watermarks, or annotations — rather than directly modifying the content within the PDF in a what-you-see-is-what-you-get (WYSIWYG) manner.
Some popular open source PHP libraries for PDF generation and manipulation are covered in detail in the following sections.
FPDF
- Enables you to generate PDFs using PHP code to define elements such as text, images, lines, and shapes at specific coordinates.
- Lightweight and doesn’t require external dependencies (except
Zlib
for compression andGD
for GIF support). - Limited HTML/CSS support.
- Good for generating documents such as invoices, certificates, and reports where layout can be controlled programmatically.
- No installation needed using a package manager such as Composer; the only requirement is to download the
fpdf.php
file from the official FPDF website and include it in your project.
Example
<?phprequire('fpdf.php');$pdf = new FPDF();$pdf->AddPage();$pdf->SetFont('Arial','B',16);$pdf->Cell(40,10,'Hello world!');$pdf->Output();?>
TCPDF
- Offers support for HTML and CSS, Unicode, and other page layout options.
- Supports multiple languages, barcodes, and QR codes.
- No external dependencies for basic functions.
- Can be more memory-intensive than FPDF for complex documents.
- Installation —
composer require tecnickcom/tcpdf
Example
<?phprequire_once('tcpdf/tcpdf.php');$pdf = new TCPDF();$pdf->AddPage();$pdf->SetFont('helvetica', '', 12);$pdf->Write(0, 'Hello world!');$pdf->Output('example.pdf', 'I');?>
Dompdf
- Library for converting HTML and CSS to PDF.
- Supports a range of CSS 2.1 features and some CSS3 features.
- Ideal for generating PDFs from webpage content or HTML templates.
- Requires either the
PDFLib PECL
extension (for better performance) or its bundledR&OS CPDF
class. - Installation —
composer require dompdf/dompdf
Example
<?phprequire_once 'vendor/autoload.php';use Dompdf\Dompdf;
$dompdf = new Dompdf();$dompdf->loadHtml('<h1>Hello world</h1><p>This is some HTML content.</p>');$dompdf->render();$dompdf->stream();?>
mPDF
- A fork of FPDF and HTML2PDF with extended features.
- Suitable for converting HTML to PDF, with support for UTF-8, CSS styles, and embedded fonts.
- Suitable for projects requiring rich formatting and non-Latin languages.
- Can be slower and consume more memory for large or complex HTML.
- Installation —
composer require mpdf/mpdf
Example
<?phprequire_once __DIR__ . '/vendor/autoload.php';$mpdf = new \Mpdf\Mpdf();$mpdf->WriteHTML('<h1>Hello world!</h1>');$mpdf->Output();?>
Snappy
- A PHP wrapper for the wkhtmltopdf command-line tool, which uses the WebKit rendering engine.
- Provides support for HTML-to-PDF conversion, as it uses a browser engine for rendering.
- Requires wkhtmltopdf to be installed on the server.
- Can have higher server load compared to pure PHP libraries.
- Installation —
composer require knplabs/knp-snappy
Example
<?phprequire_once __DIR__ . '/vendor/autoload.php';use Knp\Snappy\Pdf;
$snappy = new Pdf('/usr/local/bin/wkhtmltopdf');$snappy->generate('https://example.com', '/path/to/file.pdf');?>
FPDI
- Free PDF Parser (FPDI) is a separate library that enables you to import pages from existing PDF files into FPDF or TCPDF objects.
- It enables you to use the drawing capabilities of FPDF/TCPDF to add content (text, images, etc.) on top of existing PDF pages.
- Not a direct content editor, but allows for overlaying and appending to existing PDFs.
- Installation — Download from the FPDI website and include the files.
Example
<?phprequire_once('fpdf.php');require_once('fpdi/fpdi.php');
$pdf = new FPDI();$pdf->AddPage();$pdf->setSourceFile('existing.pdf');$tplIdx = $pdf->importPage(1);$pdf->useTemplate($tplIdx, 0, 0, 210, 297, true); // Use the imported page
$pdf->SetFont('Arial', '', 12);$pdf->SetTextColor(0, 0, 255);$pdf->SetXY(50, 50);$pdf->Write(0, 'Added text to existing PDF');
$pdf->Output('modified.pdf', 'F');?>
PDF Parser
- A library focused on extracting text and metadata from PDF files.
- Not for editing content, but can be useful if you need to read information from a PDF before generating a new one based on it.
- Installation —
composer require smalot/pdfparser
Example
<?phprequire_once __DIR__ . '/vendor/autoload.php';
use Smalot\PdfParser\Parser;
// Path to your PDF file.$pdfFilePath = 'path/to/your/document.pdf';
try { $parser = new Parser(); $pdf = $parser->parseFile($pdfFilePath);
$text = $pdf->getText();
echo ""; echo htmlspecialchars($text); echo "";
// You can also access metadata: $details = $pdf->getDetails(); echo "<h2>Metadata:</h2>"; echo ""; print_r($details); echo "";
} catch (\Exception $e) { echo 'Error parsing PDF: ' . $e->getMessage();}?>
iLovePDF API client
- While iLovePDF offers online tools, it also offers a PHP library to interact with its REST API.
- Enables you to perform various operations such as merging, splitting, compressing, rotating, and adding watermarks to PDFs.
- Not direct content editing, but provides manipulation features through its cloud service.
- Installation —
composer require ilovepdf/ilovepdf-php
While the library is free to use, the iLovePDF API might have usage limits on its free tier.
Example
Before you can use the iLovePDF API, you’ll need to sign up for an account on the website and obtain an API key.
<?phprequire __DIR__ . '/vendor/autoload.php';
use Ilovepdf\Ilovepdf;
// Replace with your iLovePDF public key.$public_key = 'YOUR_ILOVEPDF_PUBLIC_KEY';
// Path to the PDF file you want to watermark.$pdfFilePath = 'path/to/your/input.pdf';
// Path where the watermarked PDF will be saved.$outputFilePath = 'path/to/your/watermarked.pdf';
try { $ilovepdf = new Ilovepdf($public_key, null); // No secret key needed for basic operations.
$process = $ilovepdf->newTask('watermark'); $process->addFile($pdfFilePath);
// Watermark settings. $process->setText('Confidential', 'Arial', 30, '0', 45, 'center', 'middle', '50'); // Parameters: text, font, size, color (hex or name), rotation, x, y, opacity (0-100)
$process->execute(); $process->download($outputFilePath);
echo 'PDF watermarked successfully and saved to: ' . $outputFilePath;
} catch (\Ilovepdf\Exceptions\IlovepdfException $e) { echo 'iLovePDF API Error: ' . $e->getMessage();} catch (\Exception $e) { echo 'General Error: ' . $e->getMessage();}?>
Open source vs. commercial — Which PHP PDF option to use
The best approach depends on your specific needs:
- Basic viewing — Direct links or basic embedding might suffice.
- Enhanced viewing and embedding — JavaScript PDF viewer libraries offer more control.
- Generating new PDFs — FPDF, TCPDF, Dompdf, and mPDF might suffice based on complexity and HTML/CSS support.
- Structural editing — FPDI might suffice for adding content to existing PDFs, while the iLovePDF API provides manipulation features. PDF Parser helps in extracting information.
By leveraging these free and open source PHP libraries, you can integrate basic PDF viewing, creation, and manipulation capabilities into your PHP web application. However, directly editing the content of a PDF file — for example, changing existing text or moving elements around — using free open source PHP libraries is generally not straightforward. PDF is a complex format, and true content editing usually requires libraries with advanced parsing and rendering capabilities, which are often found in commercial solutions.
If you require a robust, feature-rich solution that seamlessly integrates into your PHP web application, consider Nutrient Web SDK’s PHP PDF viewer library. Our library goes beyond basic viewing, offering many powerful features right out of the box. Empower your users with:
- AI-powered document understanding — Instantly complete tasks, analyze text, and redact key information across documents using AI.
- PDF content editing and manipulation — Edit PDF content, merge/split PDFs, and generate PDFs from templates.
- Conversion tools — Convert MS Office files, convert images to PDFs, render PDFs as images, and much more.
- OCR and barcode reading — Make scans searchable, extract text from images, and read barcodes (coming soon).
- Customizable interface — Configure behavior, appearance, and tooltips, and hide specific annotation types.
- Comprehensive PDF tools — Annotate, edit, sign, fill forms, redact, and manipulate PDFs.
- Interactive forms — Create, fill, and customize interactive forms programmatically or through the UI.
- Third-party integrations — Integrate with SharePoint, Microsoft Teams, Salesforce, and more.
- Advanced text search and redaction — Search text in documents, or remove sensitive information securely.
- Measurement tools — Measure area, distance, and perimeter in PDFs.
- Bookmark and outline management — Create, edit, and remove bookmarks and structured outlines.
- Document comparison — Compare text across PDFs, analyze content changes, and detect version differences.
Try Nutrient PHP PDF viewer in under a minute
Get started instantly with the examples outlined below.
Open and view PDFs
To load PDFs, use the load
method, which takes a configuration
object as its parameter. This object specifies where the document appears, its source, your license key, and other settings. For more information, see the guides on loading a document.
Starting with version 2021.4.2, a trial license key is no longer required to evaluate the SDK. You may omit the license key or set it to an empty string. When used without a license key, the SDK applies a watermark to the output file.
<?php$licenseKey = 'YOUR_LICENSE_KEY';$documentPath = 'source.pdf';?>
<!DOCTYPE html><html><head> <title>PDF viewer</title> <script src="assets/nutrient-viewer.js"></script></head><body> <div id="nutrient" style="width: 100%; height: 100vh;"></div>
<script> PSPDFKit.load({ container: "#nutrient", document: "<?php echo $documentPath; ?>", licenseKey: "<?php echo $licenseKey; ?>" }).then(instance => { console.log("Nutrient web viewer loaded", instance); }).catch(error => { console.error("Failed to load Nutrient web viewer", error); }); </script></body></html>
Integrate AI Assistant with Nutrient Web SDK
To integrate AI Assistant with Nutrient Web SDK, pass the AI Assistant configuration and a generated JSON Web Token (JWT) to PSPDFKit.load()
. For more information, refer to our guide on AI Assistant integration with Nutrient Web SDK:
<?php$sessionId = 'your-session-id';$jwt = 'your-jwt-token';$backendUrl = 'https://your-ai-backend.com';?>
<script> PSPDFKit.load({ container: "#nutrient", document: "source.pdf", licenseKey: "<?php echo $licenseKey; ?>", aiAssistant: { sessionId: "<?php echo $sessionId; ?>", jwt: "<?php echo $jwt; ?>", backendUrl: "<?php echo $backendUrl; ?>" } });</script>
Try it in our playground or get started for free to begin integrating Nutrient PHP PDF viewer into your application.
To learn more about our library and its extensive features, refer to our blogs on how to build a PHP PDF viewer and how to build a PHP image viewer.
Invest in a solution that saves you development time and provides a superior user experience for all your PDF-related tasks.
FAQs
When should I consider a commercial PHP PDF library over free options for my application?
Consider a commercial library (such as Nutrient PDF viewer) when you need advanced features (for example, annotation, form filling, PDF editing, signing); require dedicated support and regular updates; or have strict performance and reliability requirements for crucial PDF workflows.
How do I ensure user-uploaded PDFs are safe to process with PHP libraries to prevent potential security vulnerabilities?
Sanitize user-uploaded PDFs by validating file types and sizes server-side. Be cautious when using functions that directly execute shell commands or external programs based on PDF content, as this can introduce vulnerabilities.
For collaborative document workflows requiring in-browser annotations and real-time updates, what are the typical limitations of open source PHP PDF solutions?
Open source PHP libraries primarily focus on server-side generation or basic structural manipulation. Real-time, interactive in-browser annotation and collaborative features typically require dedicated client-side components and backend integrations often found in commercial solutions (such as Nutrient PDF viewer).
When dealing with regulatory compliance and the need for secure, verifiable digital signatures within a web application, what advantages do purpose-built commercial PDF libraries often provide?
Commercial PDF libraries designed for business use (such as Nutrient PDF viewer) often incorporate advanced security features, compliance standards support (like digital signature verification and audit trails), and dedicated support for implementing legally binding electronic signatures. This can be less straightforward with open source tools and might require significant custom development.
If my application needs to redact sensitive information or programmatically manipulate specific PDF elements with high precision, how do commercial libraries compare to free alternatives?
Commercial libraries (such as Nutrient PDF viewer) offer more granular control over PDF structure and content, enabling precise programmatic manipulation and redaction capabilities that can be complex or unavailable in free alternatives. This often includes better support for PDF specifications.