How to build a Nuxt.js PDF viewer
Table of contents
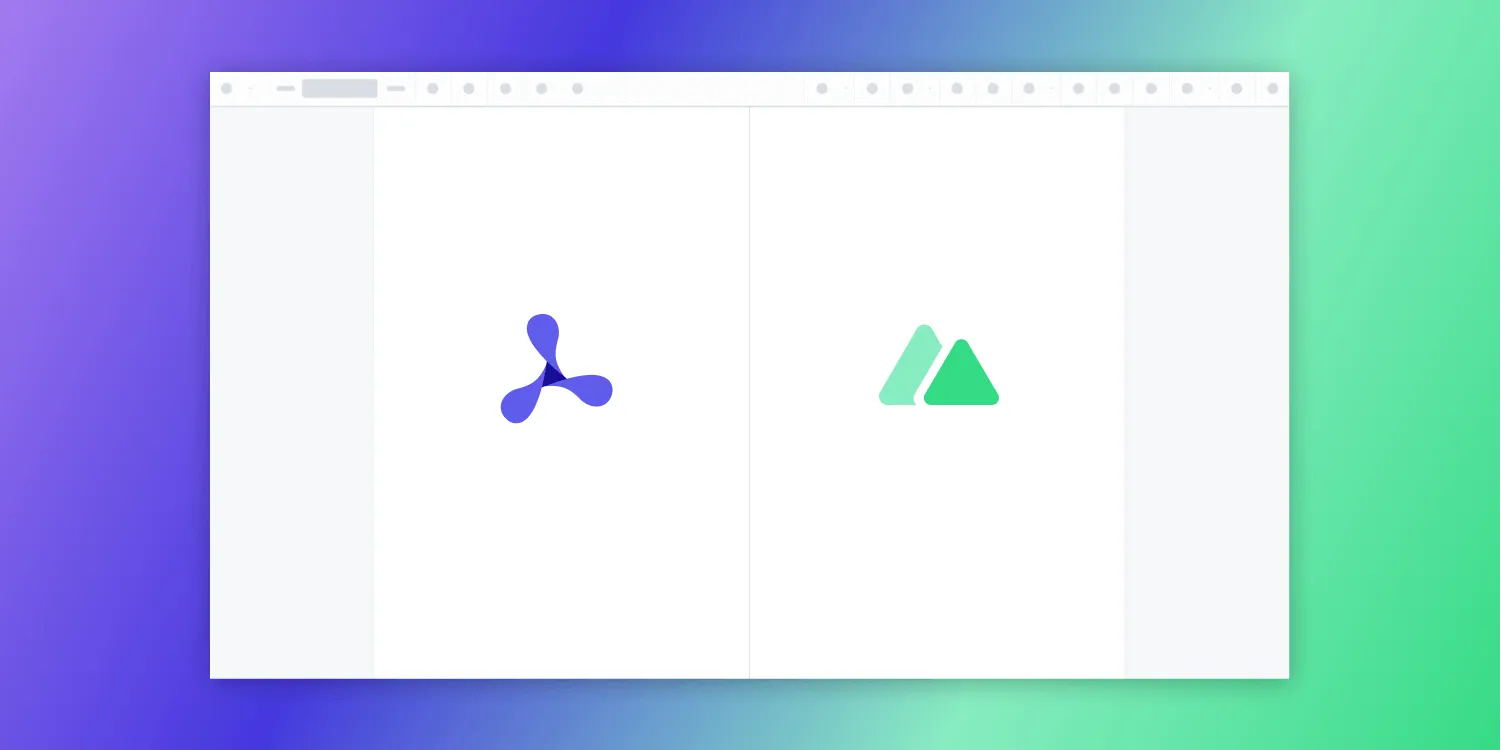
Nuxt.js(opens in a new tab) is a powerful Vue framework for building universal and statically generated applications. Nutrient Web SDK provides in-browser viewing, annotation, and editing capabilities for PDF and image documents using WebAssembly-based rendering.
What is a Nuxt.js PDF viewer?
A Nuxt.js PDF viewer lets you render and view PDF documents in a web browser without the need to download it to your hard drive or use an external application like a PDF reader.
Nutrient Nuxt.js PDF viewer
We offer a commercial PDF viewer SDK for Nuxt.js that can easily be integrated into your web application.
- A prebuilt and polished UI for an improved user experience
- 15+ prebuilt annotation tools to enable document collaboration
- Support for more file types with client-side PDF, MS Office, and image viewing
- Dedicated support from engineers to speed up integration
Example of our Nuxt.js PDF viewer
To demo our Nuxt.js PDF viewer(opens in a new tab), upload a PDF, JPG, PNG, or TIFF file by clicking Open Document under the Standalone option (if you don’t see this option, select Choose Example from the dropdown). Once your document is displayed in the viewer, try drawing freehand, adding a note, or applying a crop or an eSignature.
Requirements to get started
To get started, you’ll need:
- Node.js(opens in a new tab) (this post uses version 16.13.0)
- A package manager for installing packages — you can use npm(opens in a new tab) or Yarn(opens in a new tab)
Step 1 — Set up your Nuxt.js project
Create a fresh Nuxt.js project using the official command-line interface (CLI):
npx create-nuxt-app nutrient-pdf-viewercd nutrient-pdf-viewer
Use the default settings when prompted.
Now, open your app.vue
and replace the default Nuxt content with:
<template> <div> <NuxtPage /> </div></template>
This enables Nuxt to render pages from the /pages
directory.
Step 2 — Install the Nutrient SDK
Install the Nutrient SDK as a project dependency:
npm i @nutrient-sdk/viewer
pnpm add @nutrient-sdk/viewer
yarn add @nutrient-sdk/viewer
Step 3 — Copy the SDK assets to the public directory
Create a folder js
inside your public
directory:
mkdir -p public/js
Then copy the viewer distribution files:
cp -R ./node_modules/@nutrient-sdk/viewer/dist/ public/js/nutrient/
Ensure your structure looks like this:
/public /js /nutrient nutrient-viewer.js nutrient-viewer-lib/
To automate the copy process, add this to your package.json
scripts:
"scripts": { "copy-assets": "cp -R ./node_modules/@nutrient-sdk/viewer/dist/ public/js/nutrient/", "dev": "npm run copy-assets && nuxt dev", "build": "npm run copy-assets && nuxt build"},
Step 4 — Add a PDF file
Place a file like document.pdf
in your public/
directory. You can use our demo document as an example.
/public document.pdf
Step 5 — Create a component wrapper
The code below will create a NutrientContainer.vue
component that will dynamically load Nutrient Web SDK.
- The component receives a
pdfFile
prop to determine which document to render. - Use
mounted()
to ensure the viewer initializes after the DOM has rendered. - The SDK is loaded using
import('@nutrient-sdk/viewer')
and rendered in the.pdf-container
div.
Create a file components/NutrientContainer.vue
:
<template> <div class="pdf-container"></div></template>
<script>export default { name: 'NutrientContainer', props: { pdfFile: { type: String, required: true, }, }, mounted() { this.loadNutrient().then((instance) => { this.$emit('loaded', instance); }); }, watch: { pdfFile(val) { if (val) { this.loadNutrient(); } }, }, methods: { async loadNutrient() { import('@nutrient-sdk/viewer').then((Nutrient) => { Nutrient.unload('.pdf-container'); return Nutrient.load({ document: this.pdfFile, container: '.pdf-container', baseUrl: 'http://localhost:3000/js/nutrient/', }); }); }, },};</script>
<style scoped>.pdf-container { height: 100vh;}</style>
Step 6 — Use the component in the homepage
Create pages/index.vue
and add:
<template> <div id="app"> <label for="file-upload" class="custom-file-upload"> Open PDF </label> <input id="file-upload" type="file" @change="openDocument" class="btn" /> <NutrientContainer :pdfFile="pdfFile" @loaded="handleLoaded" /> </div></template>
<script>import NutrientContainer from '../components/NutrientContainer.vue';
export default { name: 'app', components: { NutrientContainer, }, data() { return { pdfFile: '/document.pdf', }; }, methods: { handleLoaded(instance) { console.log('Nutrient loaded:', instance); }, openDocument(event) { if (this.pdfFile && this.pdfFile.startsWith('blob:')) { window.URL.revokeObjectURL(this.pdfFile); } this.pdfFile = window.URL.createObjectURL( event.target.files[0], ); }, },};</script>
<style>#app { font-family: Avenir, Helvetica, Arial, sans-serif; text-align: center; color: #2c3e50; margin: 0;}
input[type='file'] { display: none;}
.custom-file-upload { border: 1px solid #ccc; border-radius: 4px; padding: 10px 12px; cursor: pointer; background: #4a8fed; color: #fff; font-size: 16px; font-weight: bold;}</style>
Step 7 — Run the application
Start the development server:
npm run dev
Visit http://localhost:3000
in your browser. You’ll see your PDF rendered inside the Nutrient viewer.
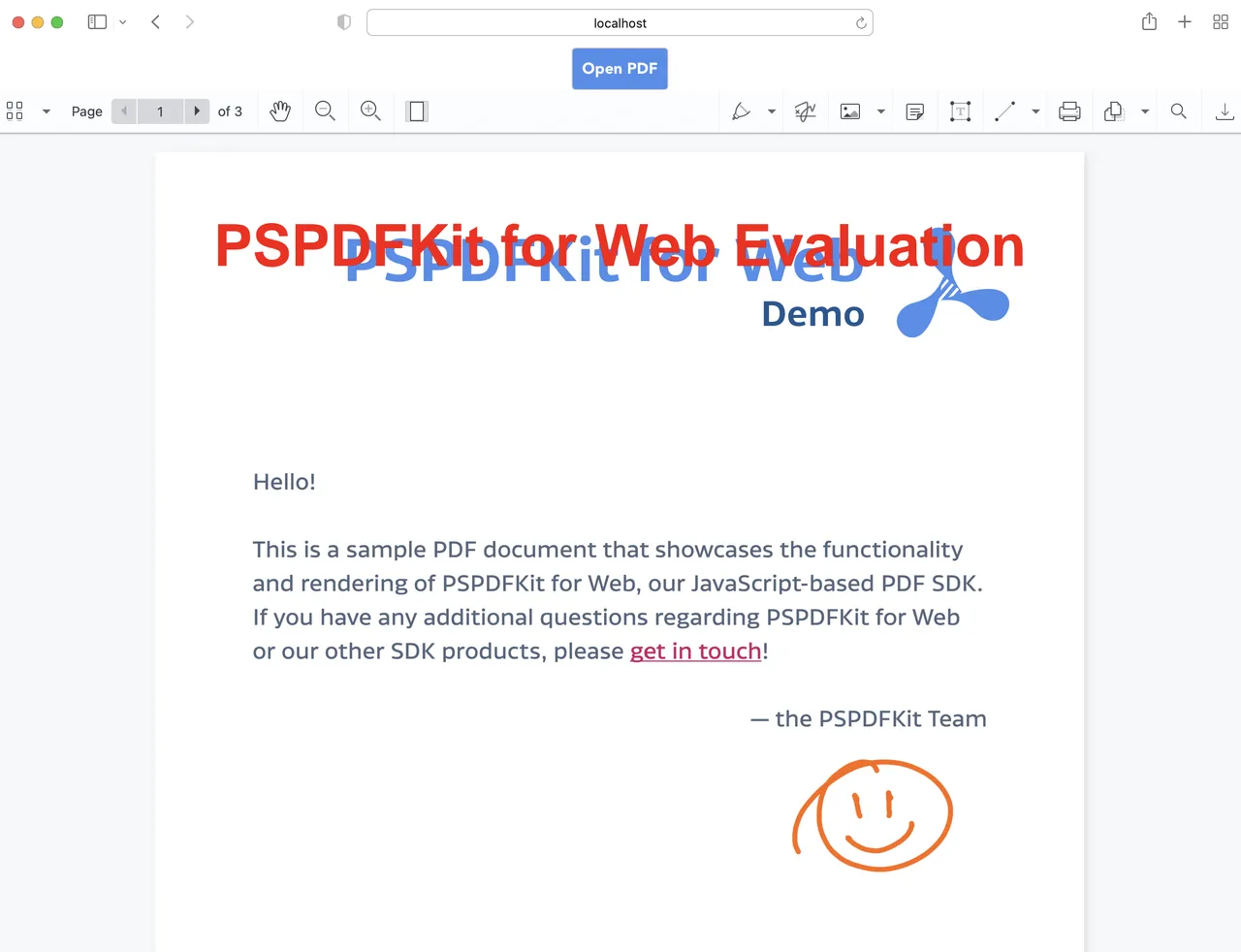
In the demo application, you can open different files by clicking the Open PDF button. You can add signatures, annotations, stamps, and more.
You can find the example on GitHub(opens in a new tab).
Adding even more capabilities
Once you’ve deployed your viewer, you can start customizing it to meet your specific requirements or easily add more capabilities. To help you get started, here are some of our most popular Nuxt.js guides:
- Adding annotations
- Editing documents
- Filling PDF forms
- Adding signatures to documents
- Real-time collaboration
- Redaction
- UI customization
Conclusion
You’ve successfully built a Nuxt.js PDF viewer using Nutrient Web SDK. This setup gives you full control over document rendering, interaction, and customization.
You can also integrate our PDF viewer using web frameworks like Angular, jQuery, Vue.js, and React.js. To see a list of all web frameworks, start your free trial. Or, launch our demo(opens in a new tab) to see our viewer in action.
FAQ
Can Nutrient SDK be used with Nuxt.js?
Yes. Nutrient Web SDK works seamlessly with Nuxt.js by loading the viewer dynamically on the client and referencing its public assets.
What file types are supported?
Nutrient supports PDFs, JPGs, PNGs, and TIFFs for viewing and annotation.
Can I upload files at runtime?
Yes. You can upload and render local files by binding to the file input and generating blob URLs.
Can I customize the appearance and tools?
Absolutely. You can tailor the toolbar, buttons, themes, and available tools through the SDK’s configuration.
Is Nutrient free to use?
Nutrient offers a free trial and commercial plans.