How to build a JavaScript image viewer
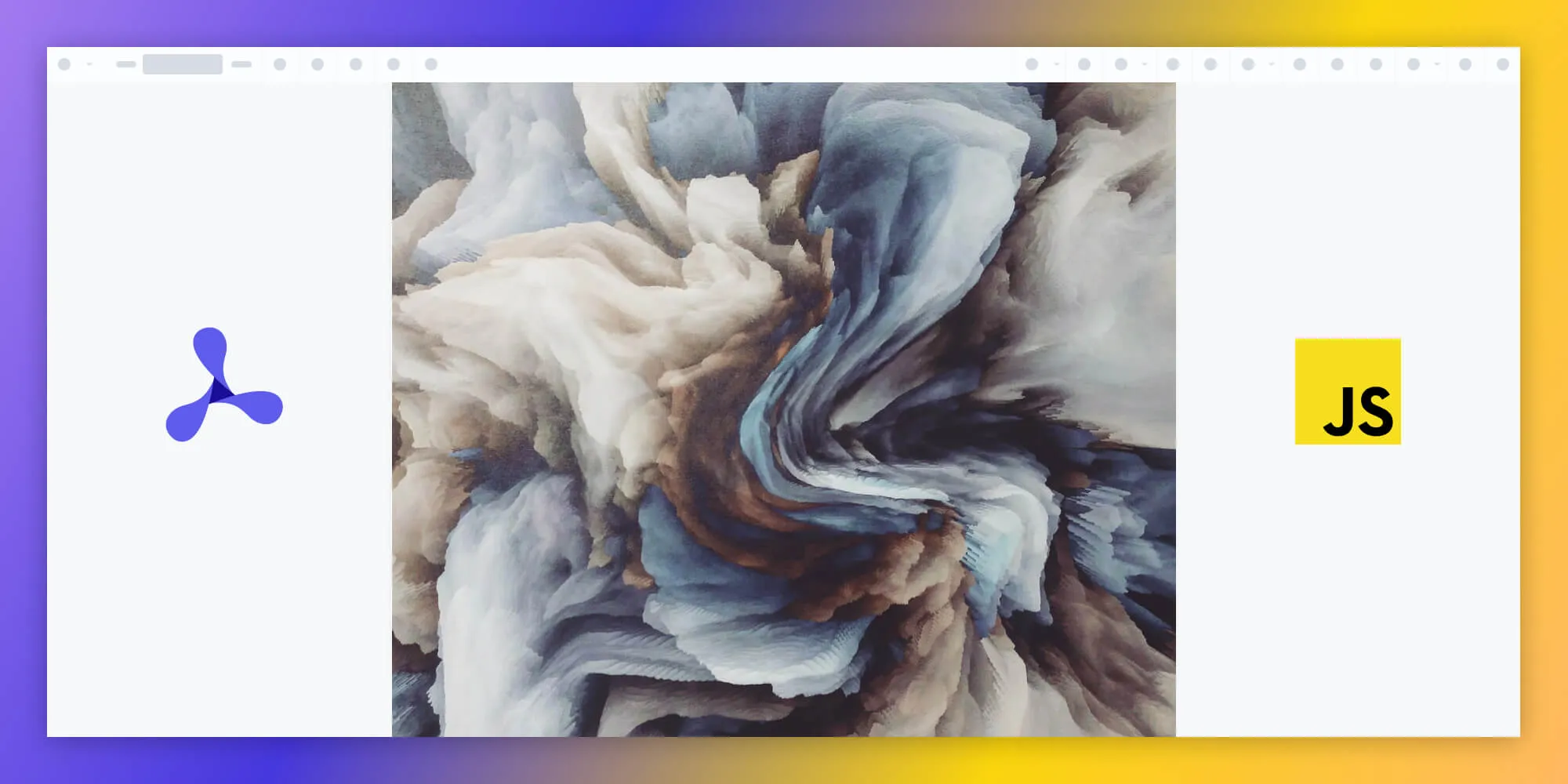
Modern web applications often need to display high-quality images and scanned documents inline, without forcing users to download them or rely on native viewers. Whether you’re building a digital dashboard, inspection workflow, or document approval system, a reliable and flexible image viewer is essential.
What is a JavaScript image viewer?
A JavaScript image viewer renders images within the browser using client-side code. It eliminates the need for downloads or third-party software, providing users with a seamless, interactive experience that supports zooming, panning, annotation, and more.
Nutrient Web SDK overview
The Nutrient SDK offers a feature-rich, modern solution for displaying and interacting with documents and images on the web. It supports:
- JPEG, PNG, TIFF, and PDF formats
- An extensible UI for annotations, zooming, cropping, signing
- No plugins or external dependencies
- Mobile and cross-browser compatibility
Example of our JavaScript image viewer
To see our image viewer in action, upload a JPG, PNG, or TIFF file by selecting Choose Example > Open Document. Upload an image and try out built-in tools like drawing, cropping, and digital signatures.
Requirements to get started
- The latest stable version of Node.js(opens in a new tab).
- A package manager compatible with npm(opens in a new tab). This guide contains usage examples for Yarn(opens in a new tab) and the npm client(opens in a new tab) (installed with Node.js by default).
Adding Nutrient to your project
- Install the
@nutrient-sdk/viewer
package via npm:
npm i @nutrient-sdk/viewer
pnpm add @nutrient-sdk/viewer
yarn add @nutrient-sdk/viewer
- Copy the required viewer artifacts to your
assets
directory:
cp -R ./node_modules/@nutrient-sdk/viewer/dist/ ./assets/
Make sure the assets
directory contains:
nutrient-viewer.js
(or an equivalent main script)- A
nutrient-viewer-lib/
directory with supporting library files
Integrating into your oroject
Include a sample document (e.g.
document.pdf
) in the public or root folder of your project. You can use our demo image as an example.Add a mounting
<div>
and a script reference to your HTML:
<div id="nutrient" style="width: 100%; height: 100vh;"></div><script type="module" src="index.js"></script>
- Import the viewer in your JavaScript entry file:
import './assets/nutrient-viewer.js';
- Initialize the viewer using
NutrientViewer.load()
:
const baseUrl = `${window.location.protocol}//${window.location.host}/assets/`;
NutrientViewer.load({ baseUrl, container: '#nutrient', document: 'document.pdf',}) .then((instance) => { console.log('Nutrient loaded', instance); }) .catch((error) => { console.error(error.message); });
Serving your website
- Install the
serve
package globally:
npm install --global serve
- Start a local server from the current directory:
serve -l 8080 .
- Open your browser and go to
http://localhost:8080
to view your website.
Output preview
If everything is set up correctly, the viewer will render your document. You can replace document.pdf
with any valid PDF to test functionality.
Adding even more capabilities
Once your viewer is set up, you can unlock powerful capabilities with just a few lines of code:
- Adding annotations
- Editing documents
- Filling PDF forms
- Adding signatures to documents
- Real-time collaboration
- Redaction
- UI customization
Conclusion
You now have a lightweight, full-featured JavaScript image viewer running in your browser using the Nutrient SDK. From simple document viewing to collaborative workflows, Nutrient provides a robust foundation for building interactive web apps.
If you want to explore more, check out our tutorials for other frameworks:
- How to build an Angular image viewer
- How to build a jQuery image viewer
- How to build a React image viewer
To see a list of all web frameworks, start your free trial. Or, launch our demo to see our viewer in action.
FAQ
What image formats are supported?
The Nutrient viewer supports JPEG, PNG, TIFF, and PDF formats. Support for other formats like BMP or GIF can be added via conversion pipelines.
Can I use this in a React or Vue app?
Yes. While this guide uses vanilla JavaScript, Nutrient integrates well with all major frontend frameworks. Framework-specific examples are available in the documentation.
Is this SDK free to use?
Nutrient is a commercial SDK. However, you can start a free trial(opens in a new tab) to evaluate the viewer and all its features.
Does this work on mobile browsers?
Yes. The SDK is fully responsive and optimized for mobile browsers, including Safari and Chrome on iOS and Android.
Can I annotate images?
Absolutely. Nutrient includes built-in support for annotations, including drawing, highlights, stamps, and text notes.
Can I use the viewer for PDF and Office files too?
Yes. The same Nutrient Web SDK used in this tutorial supports PDF, DOCX, XLSX, PPTX, and image files. Just change the file path and extension in your loadPDF()
call.
Does this viewer require a server backend?
No. Nutrient renders documents entirely in the browser using WebAssembly, so no server-side rendering is required.