How to build a jQuery PDF viewer
Table of contents
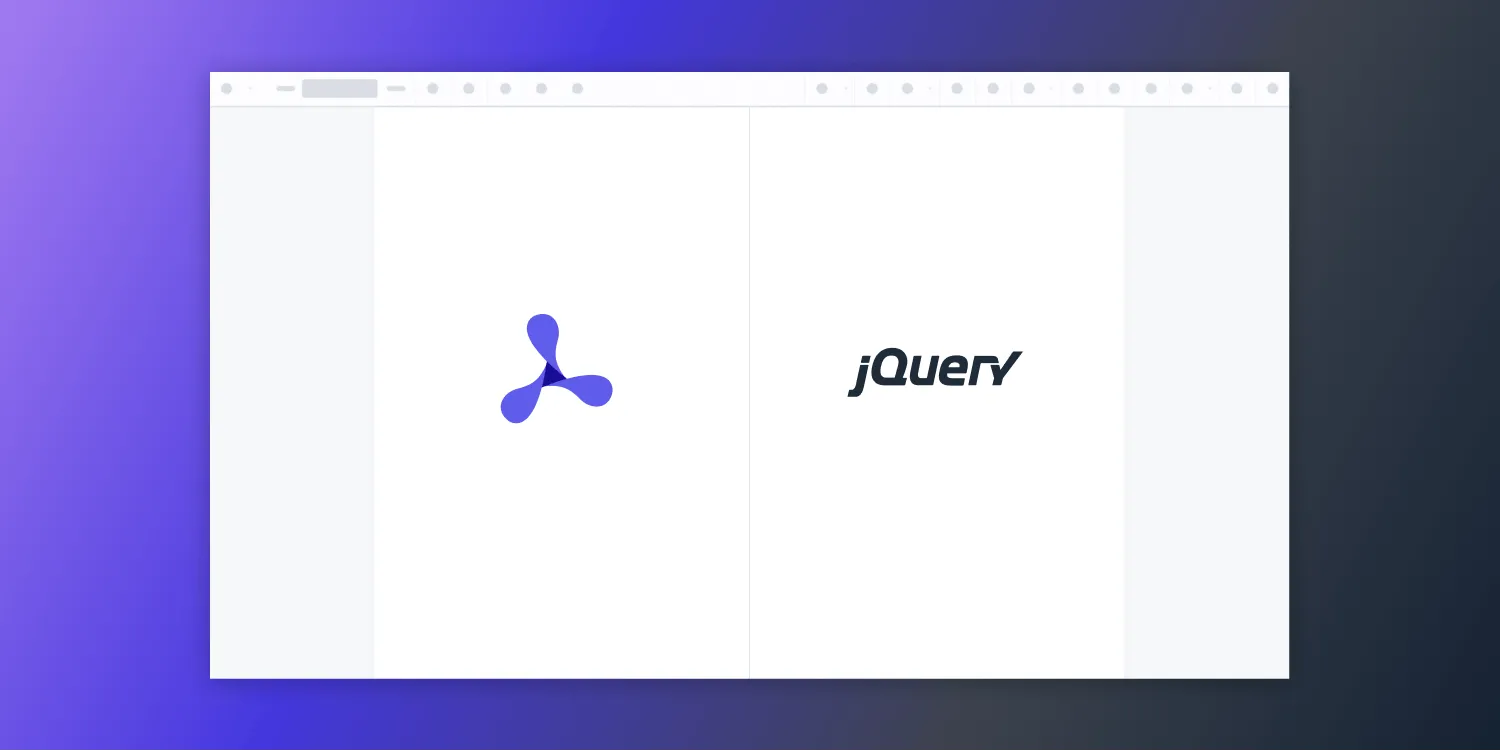
This tutorial provides a complete guide to building a browser-based PDF viewer using jQuery and Nutrient Web SDK, covering installation, setup, document rendering, and serving the viewer locally. The guide demonstrates how to integrate a lightweight JavaScript library with jQuery for modern PDF viewing capabilities, including annotation tools, document editing, signing features, and support for PDF, image, and Office file formats without requiring plugins or server rendering.
A jQuery PDF viewer lets you render and view PDF documents in a web browser without the need to download it to your hard drive or use an external application like a PDF reader.
Why use Nutrient Web SDK with jQuery
Even in a world dominated by modern frontend frameworks, jQuery remains popular for its simplicity and speed in adding interactive features. Nutrient Web SDK complements this with a polished, ready-to-use UI and deep document support, including:
- PDF, image, and Office file rendering in the browser
- Rich annotation tools, including drawing, redaction, stamping, and signing
- Built-in editing features like cropping, rotating, and page management
- A modular API for customizing the UI and viewer behavior
Preview the viewer
You can explore the live version of the viewer by uploading a PDF or selecting a demo document. The SDK runs entirely in the browser, so no plugins or server rendering are required.
What you’ll need
To get started, you’ll need:
- Node.js(opens in a new tab) — A JavaScript runtime that includes npm.
- A package manager — You can use npm(opens in a new tab), pnpm(opens in a new tab), or yarn(opens in a new tab).
- A PDF file — For testing the viewer. Place it in your project’s root directory as
document.pdf
.
Step 1 — Install the SDK
First, install Nutrient Web SDK. This pulls in all required assets, including the WebAssembly engine, fonts, and viewer UI:
npm i @nutrient-sdk/viewer
pnpm add @nutrient-sdk/viewer
yarn add @nutrient-sdk/viewer
Step 2 — Add SDK files to your project
Nutrient’s viewer works entirely in the browser but needs access to static assets (fonts, wasm files, etc.). Copy the distribution files to your assets
folder:
cp -R ./node_modules/@nutrient-sdk/viewer/dist/ ./assets/
Make sure your assets/
folder now includes:
nutrient-viewer.js
nutrient-viewer-lib/
(fonts, localization, WebAssembly runtime, etc.)
Make sure your server is configured to serve .wasm
files with the correct MIME type: Content-Type: application/wasm
.
Step 3 — Add a viewer container
In your HTML file, you’ll need a placeholder <div>
where the viewer will mount. Style it to fill the viewport:
<div id="nutrient" style="width: 100%; height: 100vh;"></div>
This is where the Nutrient viewer will embed its toolbar and document canvas.
Step 4 — Add jQuery
Nutrient works with any frontend setup, but since this tutorial uses jQuery, you’ll need to load it first. You can use the CDN version:
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
Place this above your initialization script in your HTML file.
Step 5 — Load the viewer using jQuery
Add the PDF document you want to display to your project’s directory. You can use our demo document as an example.
Now that everything’s in place, render your PDF. Create an index.js
file and import the SDK:
import "./assets/nutrient-viewer.js";
const baseUrl = `${window.location.protocol}//${window.location.host}/assets/`;
$(document).ready(function () { NutrientViewer.load({ baseUrl, container: "#nutrient", document: "document.pdf", }) .then((instance) => { console.log("Nutrient loaded", instance); }) .catch((error) => { console.error(error.message); });});
This script waits for the DOM to load and then tells Nutrient where to mount the viewer, where its assets are located, and what PDF file to load.
Load the script in your HTML
Reference index.js
in your HTML using a <script type="module">
tag:
<script type="module" src="index.js"></script>
Final HTML example
Here’s a working index.html
combining everything:
<!DOCTYPE html><html lang="en"> <head></head> <body> <div id="nutrient" style="width: 100%; height: 100vh;"></div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.0/jquery.min.js"></script> <script type="module" src="index.js"></script> </body></html>
How to run the viewer locally
You need a server to run the SDK (WebAssembly won’t load directly from file://
). One of the easiest ways to launch a local server is using serve
(opens in a new tab):
npm install --global serveserve -l 8080 .
Then open your browser to http://localhost:8080(opens in a new tab) and you’ll see your PDF rendered inside the viewer.
Adding even more capabilities
Once you’ve deployed your viewer, you can start customizing it to meet your specific requirements or easily add more capabilities. To help you get started, here are some of our most popular JavaScript guides:
- Adding annotations
- Editing documents
- Filling PDF forms
- Adding signatures to documents
- Real-time collaboration
- Redaction
- UI customization
Conclusion
You now have a fully functional, jQuery-based PDF viewer running in the browser. From rendering to annotations, Nutrient gives you the flexibility to control the entire viewing experience.
You can also deploy our vanilla JavaScript PDF viewer or use one of our many web framework deployment options like Angular, React.js, and Vue.js.
Looking for more?
FAQ
Can I display files other than PDFs?
Yes. In addition to PDFs, Nutrient Web SDK supports PNG, JPG, and TIFF image files. Just update the document
parameter accordingly.
Do I need a server to run the viewer?
Yes. Nutrient uses WebAssembly, which requires loading over HTTP or HTTPS. A simple server like serve
is sufficient for local testing.
Can I let users upload a PDF to view?
Yes. You can use a file input and dynamically reload the viewer with NutrientViewer.load()
using a blob or file URL.
Is the viewer UI customizable?
Absolutely. You can add, remove, or reposition tools, define themes, and even build your own toolbar using the SDK’s JavaScript API.
Does Nutrient support annotations and eSignatures?
Yes. Nutrient includes built-in tools for highlighting, drawing, redacting, commenting, and capturing typed or drawn signatures.