Modern PHP applications frequently require a way to render and display PDF documents directly in the browser — whether for reviewing contracts, completing forms, or verifying reports. Traditionally, PHP developers have relied on open source tools such as FPDF and TCPDF for generating PDF documents. However, these libraries don’t support client-side rendering, annotations, or real-time interaction.
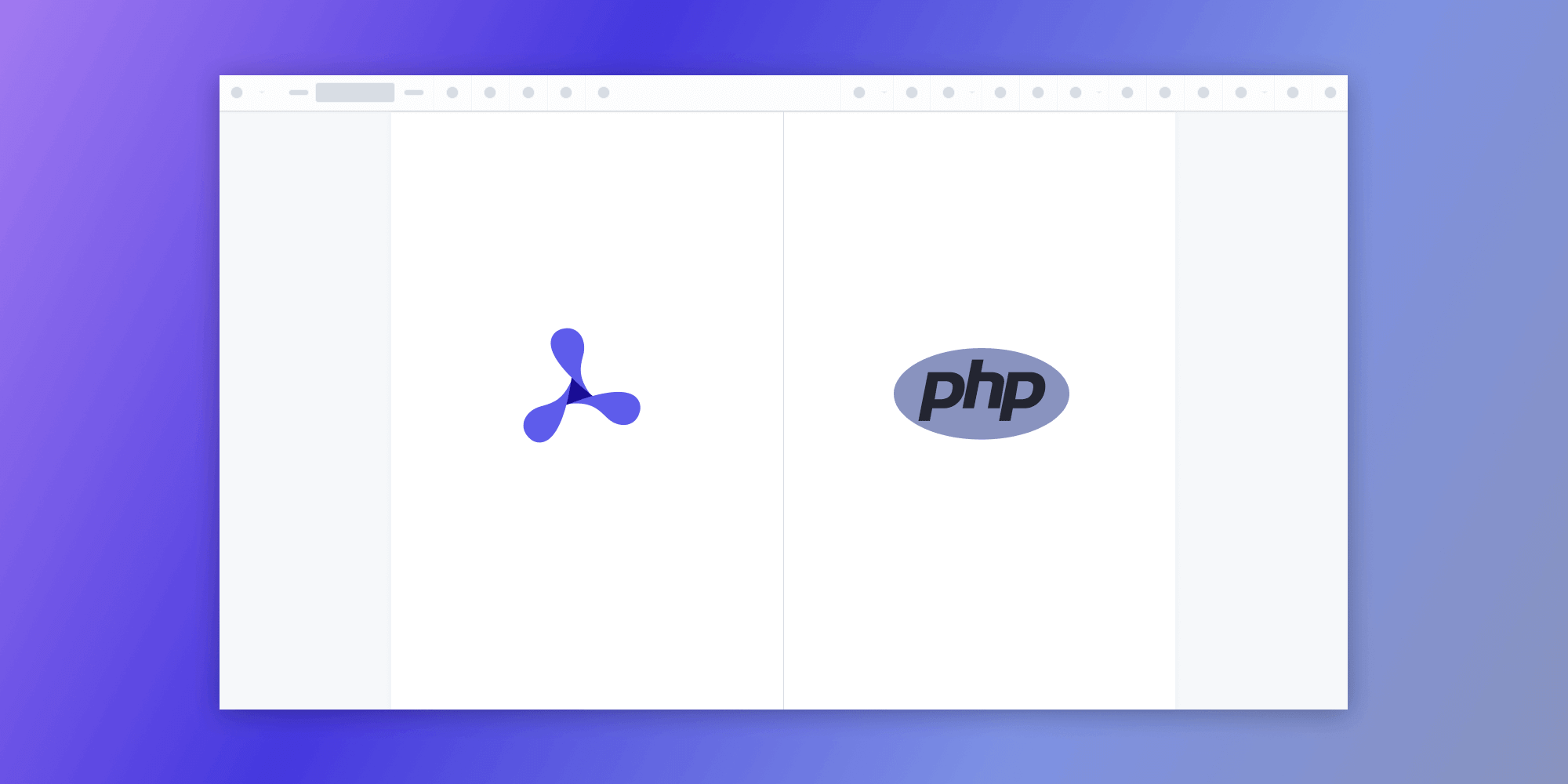
In this post, you’ll learn:
-
Why integrating a PDF viewer is valuable for PHP applications.
-
How to implement a full-featured PDF viewer using Nutrient Web SDK.
Learn how to build a Laravel PDF viewer with our Laravel PDF viewer tutorial.
What is a PHP PDF viewer?
A PHP PDF viewer uses PHP to render and view PDF documents in a web browser without the need to download it to your hard drive or use an external application like a PDF reader.
Why build a PHP PDF viewer?
PDF remains the dominant format for sharing contracts, invoices, reports, forms, and records across industries. As a result, web applications increasingly need to display PDF content directly in the browser — without forcing users to download files or switch to a third-party viewer like Adobe Acrobat.
A PHP PDF viewer enables developers to:
-
Embed documents directly in web applications, creating seamless, uninterrupted workflows.
-
Control document access and permissions through server-side logic.
-
Enhance the user experience by eliminating the need to download or open separate files.
-
Support interactive use cases like form filling, annotations, collaboration, or eSigning.
For PHP-based applications — whether internal portals, customer-facing dashboards, or CMS platforms — adding PDF viewing capabilities means users can stay inside your product while securely interacting with critical documents.
Use cases for PHP-based PDF viewers
-
Legal applications — Review, sign, or annotate contracts and agreements.
-
Healthcare systems — Display lab results, prescriptions, or intake forms.
-
Financial platforms — Show statements, invoices, and receipts.
-
Education tools — View and grade assignments or generate progress reports.
-
Enterprise portals — Preview reports, proposals, or documentation inside intranet environments.
Generating PDFs in PHP using open source libraries
Before integrating a dedicated PDF viewer into your application, you may need to generate PDF documents dynamically using PHP. Two of the most widely used open source libraries for PDF generation in PHP are FPDF and TCPDF. Both are mature and feature-rich, and they can be used to programmatically produce PDF documents such as invoices, reports, labels, certificates, or statements.
PDF generation with FPDF
FPDF is a minimalist PHP class for creating PDF files. It supports basic layout functions including text output, images, line drawing, and multi-page document creation. FPDF is lightweight, doesn’t require external dependencies, and is easy to learn.
Installation
-
FPDF doesn’t use Composer. To install, download the source files from fpdf.
-
Extract the contents into your project directory. It includes:
-
fpdf.php
-
A
font/
directory
Your structure will look like this:
fpdf-project/ ├── fpdf.php ├── font/ └── main.php
-
Now, create
main.php
and add the following code:
<?php require_once 'fpdf.php'; $pdf = new FPDF(); $pdf->AddPage(); $pdf->SetFont('Arial', 'B', 16); $pdf->Cell(40, 10, 'Hello from FPDF!'); $pdf->Output(__DIR__ . '/example-fpdf.pdf', 'F');
This script creates a simple one-page PDF and saves it as example-fpdf.pdf
in the same folder.
-
In the terminal, run:
php main.php
You’ll now see a file named example-fpdf.pdf
in your project directory. Open it in your PDF viewer to confirm that it displays the generated content.
PDF generation with TCPDF
TCPDF is a more advanced library that builds on FPDF’s capabilities. It offers additional features such as support for Unicode, right-to-left languages, HTML content, vector graphics, barcodes, and more complex layouts.
Installation
-
Ensure you have Composer installed:
composer -V
-
If not, install it from https://getcomposer.org/download/.
-
Then install TCPDF:
composer require tecnickcom/tc-lib-pdf
This creates a vendor/
folder and a composer.json
file.
Create the PHP script
Create a file called main.php
in the same directory:
touch main.php
Open it in your code editor and paste the following code:
<?php require_once 'vendor/autoload.php'; $pdf = new TCPDF(); $pdf->SetCreator(PDF_CREATOR); $pdf->SetAuthor('Your Name'); $pdf->SetTitle('TCPDF Example'); $pdf->AddPage(); $pdf->SetFont('helvetica', '', 14); $html = '<h1>Welcome to TCPDF</h1><p>This PDF was generated using TCPDF and PHP.</p>'; $pdf->writeHTML($html, true, false, true, false, ''); // Save the file to the current directory. $pdf->Output(__DIR__ . '/example-tcpdf.pdf', 'F');
From your terminal, run:
php main.php
After running the script, you’ll see example-tcpdf.pdf
in the same directory. Open it with any PDF viewer to confirm that it worked.
Choosing between FPDF and TCPDF
-
Use FPDF for simple layout needs, fast performance, and easy integration.
-
Use TCPDF when you require richer layout control, internationalization, or support for embedded HTML.
Once your PDF is generated, it can be passed to a viewer for in-browser display. The next section will demonstrate how to integrate a full-featured PDF viewer into your PHP application using Nutrient Web SDK.
Nutrient PHP PDF viewer
Nutrient PHP PDF viewer is a powerful JavaScript-based viewing library designed for seamless integration into PHP web applications. As part of the Nutrient Web SDK, it provides high-performance, in-browser PDF rendering with support for advanced interactions — no external plugins or downloads required.
Built with flexibility and enterprise needs in mind, it allows you to embed professional-grade document viewing and collaboration tools directly within your PHP stack.
-
Customizable UI — Adapt toolbars, themes, buttons, and layouts to create a seamless user experience that fits your brand guidelines.
-
Client-side rendering — All document rendering happens in the browser — ensuring fast performance, better scalability, and reduced server load.
-
Multi-format support — View not only PDFs, but also Office documents (DOCX, XLSX, PPTX) and image files (JPG, PNG, TIFF) using a single viewer.
-
Mobile-friendly viewer — Nutrient’s responsive interface is optimized for all screen sizes, ensuring usability across phones, tablets, and desktops.
-
Accessibility features — Built-in ARIA roles, keyboard navigation, and screen reader compatibility make the viewer compliant with modern accessibility standards.
-
Cross-browser compatibility — Fully supported across all major modern browsers, including Chrome, Firefox, Safari, and Microsoft Edge.
-
Extendable with annotations and more — Add support for markup, form filling, eSignatures, redaction, collaboration, and editing tailored to your application’s needs.
Example of our PHP PDF viewer
To demo our PHP PDF viewer, upload a PDF, JPG, PNG, or TIFF file by clicking Open Document under the Standalone option (if you don’t see this option, select Choose Example from the dropdown). Once your document is displayed in the viewer, try drawing freehand, adding a note, or applying a crop or an eSignature.
Requirements to get started
To get started, you’ll need:
- PHP
You can install PHP via XAMPP, MAMP, or Homebrew.
Integrating into your project
-
Add the PDF document you want to display to your project’s directory. You can use our demo document as an example.
-
Add an empty
<div>
element with a definedheight
andwidth
to where Nutrient will be mounted:
<div id="nutrient" style="width: 100%; height: 100vh;"></div>
-
Include the Nutrient viewer via CDN in your PHP page:
<script src="https://cdn.cloud.pspdfkit.com/[email protected]/nutrient-viewer.js"></script>
-
Initialize the Nutrient viewer using JavaScript inside your PHP page:
<script> NutrientViewer.load({ container: "#nutrient", document: "document.pdf" }) .then(function(instance) { console.log("Nutrient loaded", instance); }) .catch(function(error) { console.error(error.message); }); </script>
You can see the full index.php
file (which is just a plain HTML file):
<!DOCTYPE html> <html> <head> <title>My App</title> <meta name="viewport" content="width=device-width, initial-scale=1.0, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no" /> </head> <body> <!-- Element where Nutrient will be mounted --> <div id="nutrient" style="width: 100%; height: 100vh;"></div> <!-- Load Nutrient Viewer from CDN --> <script src="https://cdn.cloud.pspdfkit.com/[email protected]/nutrient-viewer.js"></script> <script> NutrientViewer.load({ container: "#nutrient", document: "document.pdf" // Your PDF file }) .then(function(instance) { console.log("Nutrient loaded", instance); }) .catch(function(error) { console.error("Failed to load Nutrient:", error.message); }); </script> </body> </html>
Serving your website
-
Go to your terminal and run a server with this command:
php -S 127.0.0.1:8000
-
Navigate to http://127.0.0.1:8000/index.php to view the website.
Adding even more capabilities
Once you’ve deployed your viewer, you can start customizing it to meet your specific requirements or easily add more capabilities. To help you get started, here are some of our most popular PHP guides:
- Adding annotations
- Editing documents
- Filling PDF forms
- Adding signatures to documents
- Real-time collaboration
- Redaction
- UI customization
Conclusion
A PHP PDF viewer lets you display and interact with documents directly in the browser, with no downloads or plugins required. In this guide, you learned how to generate PDFs using FPDF and TCPDF, as well as how to integrate a full-featured viewer with Nutrient Web SDK for seamless in-browser rendering, annotation, and form handling.
You can also integrate our JavaScript PDF viewer using web frameworks like Angular, Vue.js, and React.js. To see a list of all web frameworks, start your free trial. Or, launch our demo to see our viewer in action.