Search text in PDFs using JavaScript
Nutrient Web SDK provides a robust full-text search functionality for PDFs. It counts the occurrences of a search term, allows seamless navigation through the results, and highlights all matches directly in the document. Essentially, it operates much like your browser’s search feature. But what if you need a customized PDF search using JavaScript to behave differently or meet specific requirements?
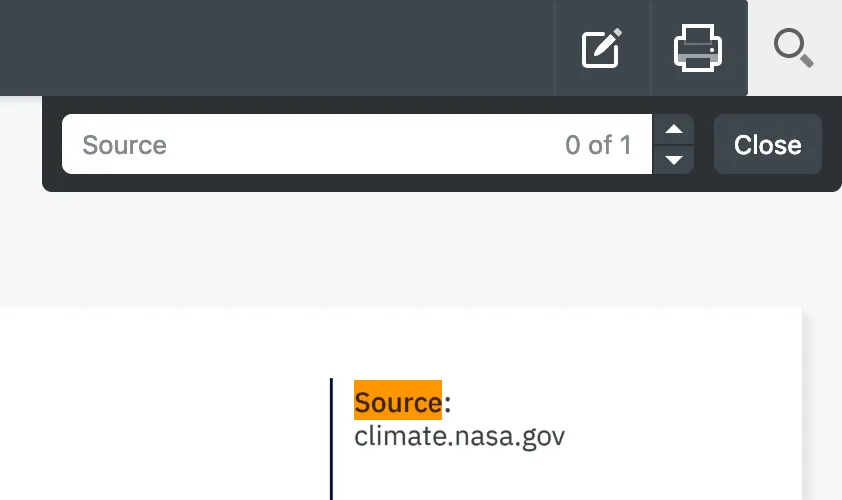
Customizing PDF search with JavaScript
Nutrient Web SDK enables developers to customize search behavior by hooking into search queries via the search.termChange
event, which triggers on every text change in the search field:
let lastSearchTerm = "";
instance.addEventListener("search.termChange", async (event) => { // Opt out from the default implementation. event.preventDefault();
const { term } = event; // Update the search term in the search box. Without this line, // the search box would stay empty. instance.setSearchState((state) => state.set("term", term)); lastSearchTerm = term;
// Perform a custom search for the term. const results = await customSearch(term, instance);
// Our results could return in a different order than expected. // Let's make sure only results matching our current term are applied. if (term !== lastSearchTerm) { return; }
// Finally, we apply the results. Note that you can also modify // the search state first and then pass the new state // to `instance.setSearchState`. const newState = instance.searchState.set("results", results); instance.setSearchState(newState);});
For instance, if you want your PDF search results to match only whole words, you can achieve this by leveraging the customSearch
function. This function utilizes Nutrient Web SDK’s instance.search
API, requiring instance
as an argument. After executing the default search, results can be filtered to display only whole-word matches, ensuring a more precise customized PDF search experience:
async function customSearch(term, instance) { // We would get an error if we called `instance.search` with a term of // 2 characters or less. if (term.length <= 2) { return PSPDFKit.Immutable.List([]); }
// Let's take the results from the default search as the foundation. const results = await instance.search(term);
// We only want to find whole words that match the term we entered. const filteredResults = results.filter((result) => { const searchWord = new RegExp(`\\b${term}\\b`, "i"); return searchWord.test(result.previewText); });
return filteredResults;}
Highlighting custom search results
You can highlight custom search results using one of the following:
Highlighting custom PDF search results with highlight annotations
To enhance your customized PDF search experience, you can visually emphasize search results with highlight annotations. Follow the steps below:
- Use the
search
API to perform a search across the entire PDF or a specific page range. - Use the
create
API to generate highlight annotations around the search results, ensuring users can easily locate key information.
The code below highlights the word hello
on all the pages of a PDF document:
const results = await instance.search("hello");
const annotations = results.map((result) => { return new PSPDFKit.Annotations.HighlightAnnotation({ pageIndex: result.pageIndex, rects: result.rectsOnPage, boundingBox: PSPDFKit.Geometry.Rect.union(result.rectsOnPage) });});instance.create(annotations);
Highlighting custom PDF search results with custom overlay items
For a more dynamic approach to emphasizing PDF search results, you can use custom overlay items. Follow the steps below:
- Perform a search across the PDF or specific pages using the
search
API. - Surround the search results with custom overlay items to add a layer of interactivity and style to the document.
The code below highlights the word hello
on all the pages of a PDF document:
instance .search("hello") .then((results) => { results.toJS().forEach((result, i) => { const div = document.createElement("div"); div.style.backgroundColor = "#808000"; div.style.mixBlendMode = "multiply"; div.style.opacity = 0.5; div.style.width = result.rectsOnPage[0].width + "px"; div.style.height = result.rectsOnPage[0].height + "px"; const item = new PSPDFKit.CustomOverlayItem({ id: "overlay" + i, node: div, pageIndex: result.pageIndex, position: new PSPDFKit.Geometry.Point({ x: result.rectsOnPage[0].left, y: result.rectsOnPage[0].top }) }); instance.setCustomOverlayItem(item); }); }) .catch(console.log);
Implementing a custom PDF search functionality
Take your customized PDF search experience to the next level by implementing your own search logic. Your customSearch
function could range from a simple local implementation, like the one in the example above, to a robust query sent to a large search data center. The key is to return the search results in the correct format for the searchState
.
Ensure your results are provided as a PSPDFKit.Immutable.List
of PSPDFKit.SearchResult
for seamless integration with Nutrient Web SDK’s customized PDF search capabilities.
Additional information
For more information, see our detailed API documentation pages:
PSPDFKit.SearchTermChangeEvent
PSPDFKit.SearchState
PSPDFKit.Instance.setSearchState
PSPDFKit.SearchResult